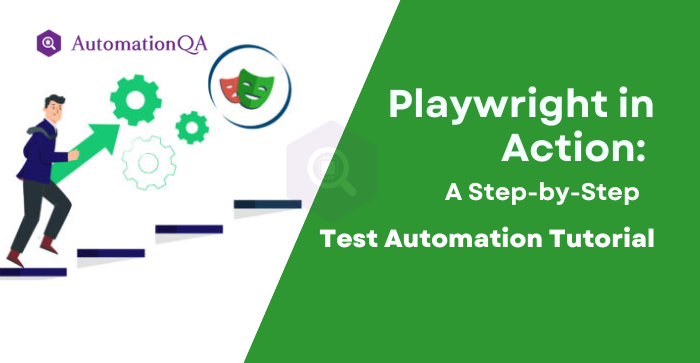
Test automation has become integral to the software development lifecycle for several reasons. It facilitates developers’ early detection of problems, expediting the entire development cycle. Another significant advantage that automation testing brings to the table is consistency and accuracy. Automation testing can be costly to set up initially, but it is worthwhile in the long term. Nonetheless, many businesses need help selecting the best testing instrument for their development procedure.
Are you experiencing the same difficulties choosing the best automation tool? However, with our strategic approach, the selection process will be manageable. The playwright test is one of today’s latest and most powerful tools. It offers developers a robust toolkit for automating interactions with the applications across various platforms. This blog will walk you through this tool and provide a step-by-step process for writing your first test script in Playwright. So, let’s get into the details to help you master automation testing using Playwright.
Playwright Essential Components and Processes
Playwright is designed to deliver a robust, flexible, high-performance browser automation solution. Its multi-browser support, isolated browser contexts, and comprehensive API make it a top choice for tasks like web scraping, automated testing, and other browser-driven operations. The architecture of Playwright Test Automation guarantees the dependability, effectiveness, and ease of maintenance of tests and scripts.
Client Side (Automation Scripts)
Languages Supported: Playwright has native support for TypeScript, JavaScript, Java, Python, and C# for developing automation scripts. Because of this flexibility, developers can work in the language that best suits them.
Test Script Creation: It allows users to create test scripts in their chosen language. These scripts include specific test cases, interface commands, and assertions. JSON is commonly used for configuration and data exchange to simplify managing test data.
WebSocket Connection
Handshake: When a test automation script is executed in Playwright, it initiates a WebSocket connection to the server running on Node.js. An initial handshake is involved in establishing secure communication.
Full Duplex Communication: After establishing the connection, the client and the server maintain a bidirectional communication channel. This channel sends commands to the browser and receives real-time events or responses.
Open and Persistent Connection: The WebSocket connection stays active throughout the testing session, enabling constant communication between the test scripts and the browser. It ensures smooth test execution and immediate feedback on actions performed.
Connection Closure: When the Playwright test run is complete, the session may end when the client or the server cuts the connection.
Server Side (Node.js)
Node.js Server: A Node.js application manages the server side of Playwright. This server manages the communication between the client scripts and the browsers to guarantee that orders are correctly transmitted and carried out.
Handling Commands and Events: The Node.js server takes in commands from the client, interprets them, and then provides the browsers with the necessary instructions. It also listens for events from the browser, such as page loads or element interactions, and relays them back to the client, enabling real-time test validation.
Browser Automation (CDP and CDP+)
CDP (Chrome DevTools Protocol): It is used by Playwright test automation to interface with Chromium-based browsers. CDP handles rendering, browser management, and network processes, which are critical for tasks like page rendering, session management, and network traffic monitoring.
CDP+: In addition to CDP, Playwright supports Firefox and WebKit (Safari) through similar protocols. It ensures a consistent API across different browsers, allowing developers to write cross-browser tests quickly. Each browser process, including rendering, browser management, and network interactions, is managed to simulate user behavior and interactions accurately.
By integrating these components, Playwright offers a comprehensive framework that supports automated testing across multiple browsers and ensures that tests are executed efficiently and reliably.
Prerequisites To Set Up Playwright Test
Install NodeJS
NodeJS is a JavaScript runtime essential for installing and managing dependencies, running scripts, and setting up the development environment for Playwright. The latest version can be installed from the official NodeJS website.
Install Visual Studio Code
Visual Studio Code (VS Code) supports various programming languages and tools, including Playwright. It features integrated terminals, debugging, and extensions that enhance the development process, making it the preferred choice for writing and running Playwright tests.
Step-by-Step Process For Setting Up a Project Using Playwright Automation Testing
Step 1: Installing Playwright
Before writing tests, you must install Playwright, available as a Node.js package. If you haven’t installed Node.js, download it from the official Node.js website. After installing Node.js, launch a terminal window and type the following command to install Playwright along with the required browser binaries:
npm init playwright
This command installs Playwright and initializes a new Node.js project with the required browsers.
Step 2: Writing Your First Test
After configuring the Playwright testing tool, you can create your first test. The Playwright API is easy to use, facilitating test creation.
Create a Test File: Make a new file with the name example. In your project directory, locate spec.js.
Write a Simple Test: The code below should be copied and pasted into your example. The file spec.js:
const { test, expect } = require('@playwright/test');
test('has title', async ({ page }) => {
await page.goto('https://example.com');
await expect(page).toHaveTitle('Example Domain');
});
This test navigates to example.com and checks if the page title is “Example Domain”.
Step 3: Running Your Test
Playwright’s test dashes and may be initiated simply from the command line.
Run the Test: Use the following command in your terminal to execute the test:
npx playwright test
Check the Results: The playwright will run the test and display the results in the terminal. If everything is set up correctly, you should see a passing test result.
Step 4: Advanced Test Configuration
As you gain more experience using Playwright, you can investigate advanced capabilities to improve your testing environment.
Browser Contexts: Playwright lets you create isolated browser contexts, which is helpful for simultaneously testing multiple independent sessions.
Auto-Waiting: Playwright eliminates the need for explicit waits in your tests by allowing pieces to become available automatically.
Parallel Testing: Test execution can be accelerated by configuring Playwright to perform tests.
Cross-Browser Testing: Playwright allows a test automation company to run tests across browsers (Chromium, Firefox, WebKit) to ensure consistent platform behavior.
Step 5: Integrating with CI/CD Pipelines
Playwright integration with CI/CD pipelines is essential for development methods that involve continuous testing.
Popular CI Tools: Playwright works well with CircleCI, Jenkins, GitHub Actions, and other CI technologies. Enable Playwright to execute tests automatically on your continuous integration workflow code changes.
Configuring CI/CD: To execute Playwright tests effectively, make sure your continuous integration environment has Node.js and the required browser binaries installed.
Execute the Test Cases Locally
Playwright test cases can be executed in either headless or headed mode. The former requires opening the browser window for the tests to run locally. The steps to perform Playwright tests in both modes are listed below.
Test Cases in Headed Mode
When in headed mode, a visible browser window displays the test cases that are being executed.
1. Start by opening the terminal.
2. Locate the directory containing your test suites.
3. To run the tests in headed mode, run the following command:
```bash
npx playwright test --ui
```
Or, if you're using Yarn:
```bash
yarn playwright test --ui
```
4. The Test Runner UI will open When automation testing companies run the command. Click on the `.spec.ts` file, such as `testGrid.spec.js`, to execute the test case in headed mode.
5. Test Execution
- When you click `testGrid.spec.js`, the test case will execute in a visible browser window.
- Once the test case passes successfully, you will see a detailed execution log.
Screenshots of the test case execution can be captured during this process for further analysis.
Test Cases in Headless Mode
Playwright runs in headless mode by default, a background-only mode of operation for the browser.
1. Run this command to execute the playwright automation testing in headless mode:
```bash
npx playwright test
```
Or, if you're using Yarn:
```bash
yarn playwright test
```
2. You can also specify a particular test file like this:
```bash
npx playwright test tests/testGrid.spec.js
```
3. Test Execution
The test case will execute in the background, and once it is complete, Playwright will provide the test results in the terminal.
You can see that the test case has passed the specified browser (e.g., Chrome).
4. Generate an HTML Report
After the test execution, you can generate a report by running:
```bash
npx playwright show-report
```
This command will open an HTML report detailing the results of your test cases.
Playwright Testing Tool Best Practices
Leverage Playwright’s Assertions
Use Playwright’s built-in assertions like `toBeVisible`, `toHaveText`, and `toHaveURL` to validate the application state after user interactions. It improves code maintainability and readability.
“`typescript
test(‘login form is displayed’, async ({ page }) => {
await page.goto(‘https://your-app.com/login’);
await expect(page.locator(‘#username’)).toBeVisible();
await expect(page.locator(‘#password’)).toBeVisible();
});
“`
Utilize Playwright’s Wait Strategies (H3)
Ensure elements are ready for interaction using wait functions like `waitForSelector` or `waitForNavigation` to ensure elements are ready for interaction, which enhances test reliability.
```typescript
await page.waitForSelector('#submit-button');
```
Keep Tests Independent
One best practice that a test automation company must adopt is to ensure that each test functions independently using its data, cookies, and local storage. Use hooks like `beforeEach` and `afterEach` to set up and tear down test environments.
```typescript
test.beforeEach(async ({ page }) => {
await page.goto('https://your-app.com/login');
await page.fill('#username', 'user1');
await page.fill('#password', 'password123');
await page.click('button[type="submit"]');
});
```
Take Advantage of Playwright’s Tooling
Automation testing companies employ Codegen to generate test code from recorded browser actions, Trace Viewer to analyze failures, and Playwright Inspector for visual debugging.
Use Chaining and Filtering
Combine locators to focus on specific page sections or filter by text or another locator.
```typescript
await page
.getByRole('listitem')
.filter({ hasText: 'Product 2' })
.getByRole('button', { name: 'Add to cart' })
.click();
```
Final Words
The Playwright test automation framework supports multiple browsers and platforms, making it a robust testing tool. It offers rich APIs for interaction and validation, making it ideal for developers and testers. Following the processes and best practices above, you can develop reliable, efficient, and scalable automated tests that improve your applications’ overall quality and performance. As a result, using Playwright can provide a more reliable user experience while streamlining testing procedures and lowering manual labor.
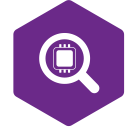
AutomationQA
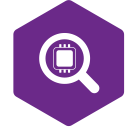
Latest posts by AutomationQA (see all)
- Step-By-Step Guide To Writing Reliable Scripts With Playwright Test - September 10, 2024
- Your Ultimate Step-By-Step Guide To Selenium Automation Testing 2024 - September 3, 2024
- Functional Testing Demystified: A Deep Dive Into Testing Types - August 16, 2024