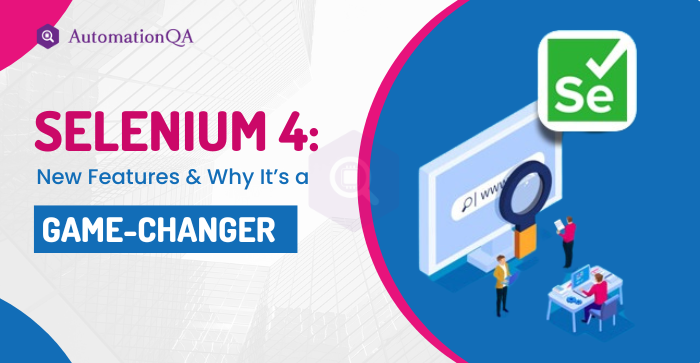
Selenium has long been the backbone of web application testing, enabling developers and QA engineers to automate repetitive tasks and ensure seamless user experiences. Introduced in 2004 as Selenium Core, it has evolved significantly over the years, with Selenium WebDriver and Selenium Grid transforming how Test Automation Companies approach browser-based testing.
With the release of Selenium 4, the framework has taken a giant leap forward, integrating modern features like W3C WebDriver compliance, advanced relative locators, and Chrome DevTools Protocol. What sets Selenium 4 apart?
Beyond just new features, it redefines the way Test Automation Companies and QA teams approach automation. This article goes beyond the surface to explore the architecture changes, advanced features, and practical implementations that make Selenium 4 a game-changer. For Selenium Automation Companies, it’s not just an update – it’s a leap into the future of web automation.
A Quick Overview of Selenium’s Journey
- 2004: Selenium Core launched as a JavaScript framework.
- 2007: Selenium IDE and WebDriver introduced.
- 2011: Selenium 2 combined WebDriver and Selenium Core.
- 2016: Selenium 3 added support for modern browsers.
- 2021: Selenium 4 redefines test automation with advanced capabilities.
Important Architecture Changes in New Selenium 4?
The most notable architectural enhancement in Selenium 4 is its shift to the W3C WebDriver Protocol. This standardization resolves many inconsistencies and improves cross-browser compatibility. Here’s why it matters:
- Standardization: Replaces the outdated JSON Wire Protocol, leading to more consistent browser behavior.
- Enhanced Stability: Minimizes issues caused by browser-specific implementations.
- Streamlined Communication: Improves communication between the browser and WebDriver, ensuring reliable automation workflows.
In Selenium 3, commands were translated from JSON Wire Protocol to WebDriver, causing compatibility issues. Selenium 4 eliminates this extra layer, ensuring direct communication with browsers.
from selenium import webdriver
from selenium.webdriver.common.by import By
# Initialize WebDriver with W3C Protocol
driver = webdriver.Chrome()
driver.get("https://example.com")
print("Page Title:", driver.title)
driver.quit()
What are the New Selenium 4 Features
Let’s have a look the new features in Selenium 4 and see how different they are from Selenium 3:
W3C WebDriver Protocol
The shift to W3C compliance is one of the most significant updates in Selenium 4. This ensures:
- Standardized Communication: Reduces compatibility issues across browsers.
- Improved Stability: Minimizes errors caused by encoding and decoding requests.
- Future-Proofing: Ensures seamless integration with modern browsers.
Code Example:
from selenium import webdriver
driver = webdriver.Chrome()
driver.get("https://example.com")
print("Page Title:", driver.title)
driver.quit()
Enhanced Selenium Grid
Selenium Grid 4 introduces a redesigned architecture that simplifies distributed testing:
- Hub-Node Simplification: Automatically acts as both hub and node.
- Docker Support: Spin up containers for lightweight testing environments.
- Scalable Deployments: Kubernetes compatibility for large-scale operations.
- Improved UI: User-friendly interface for monitoring sessions and logs.
Command to Start Standalone Grid:
java -jar selenium-server-4.x.x.jar standalone
Relative Locators
Relative locators make it easier to identify elements as per their spatial relationship to different elements:
- above(): Locates an element above a specified one.
- below(): Locates an element below a specified one.
- toLeftOf(): Locates an element to the left of another.
- >toRightOf(): Locates an element to the right of another.
- near(): Locates an element within a specified pixel range.
Code Example:
from selenium.webdriver.support.relative_locator import locate_with
driver.get("https://example.com") input_field = driver.find_element(By.ID, "username") login_button = driver.find_element(locate_with(By.TAG_NAME, "button").below(input_field)) login_button.click()
Chrome DevTools Protocol Integration
The DevTools Protocol allows direct interaction with browser features like:
- Network Monitoring
- Performance Profiling
- Console Log Retrieval
Code Example for Network Requests:
from selenium.webdriver.common.devtools.v85.network import Network
devtools = driver.get_devtools_session() devtools.send(Network.enable())
def log_request(request): print("Request URL:", request['url'])
devtools.on(Network.requestWillBeSent, log_request) driver.get("https://example.com") driver.quit()
Multi-Window and Tab Management
Selenium 4 allows seamless switching between multiple tabs and windows:
Code Example:
driver.switch_to.new_window('tab')
driver.get("https://example.com/new-tab")
driver.switch_to.new_window('window')
driver.get("https://example.com/new-window")
Element-Level Screenshots
Capture screenshots of specific elements instead of the entire page:
Code Example:
from selenium.webdriver.common.by import By
element = driver.find_element(By.ID, "example") screenshot = element.screenshot_as_file("element.png")
Improved Selenium IDE
The new Selenium IDE offers:
- Cross-Browser Support: Record and playback tests on Chrome, Firefox, and Edge.
- Parallel Execution: Run multiple test suites simultaneously.
- Detailed Reporting: Track execution status and errors.
Difference Between Selenium 3 and Selenium 4
Feature | Selenium 3 | Selenium 4 |
---|---|---|
Protocol | JSON Wire Protocol | W3C WebDriver Protocol |
Relative Locators | Not Available | Introduced for easier element search |
Selenium Grid | Complex setup | Simplified architecture |
DevTools Integration | Not Available | Chrome DevTools Protocol Support |
Improved Documentation | Limited and fragmented | Comprehensive and well-organized |
Window and Tab Management | Limited functionality | Full support for managing tabs and windows |
Performance Testing Support | No direct support | Built-in network and performance profiling through CDPBuilt-in network and performance profiling through CDP Built-in network and performance profiling through CDP |
Backward Compatibility | Fully backward-compatible | Minor adjustments needed for deprecated methods |
How to Upgrade to Selenium 4
Upgrading to Selenium 4 requires careful planning and execution to ensure compatibility with your existing frameworks and test cases. Here’s a step-by-step guide:
Step 1: Update Your Selenium Dependency
Begin by upgrading the Selenium library to the latest version. Use the following command:
pip install selenium --upgrade
This ensures you have the new features, security setup, and bug fixes offered by Selenium 4.
Step 2: Replace Deprecated Methods
Selenium 4 has deprecated several methods used in Selenium 3. For example, the find_element_by_* methods have been replaced with a more standardized approach using the By class.
Before (Selenium 3):
driver.find_element_by_id("username")
After (Selenium 4):
from selenium.webdriver.common.by import By
driver.find_element(By.ID, "username")
Make sure to update all instances of these deprecated methods in your test scripts.
Step 3: Adapt to W3C WebDriver Protocol
Ensure your framework supports the W3C WebDriver Protocol. Selenium 4 eliminates the need for encoding/decoding of requests, which simplifies browser communication but may require updates to:
- Custom WebDriver implementations
- Browser configurations
- Third-party integrations
Test your framework thoroughly to verify compatibility and performance.
Step 4: Test Your Selenium Grid Configuration
If you’re using Selenium Grid, update your configuration to leverage the enhanced architecture of Selenium 4. This includes:
- Setting up Docker containers for efficient test environments.
- Using Kubernetes for scalability.
- Testing the new hub-node auto-detection functionality.
Command Example:
java -jar selenium-server-4.x.x.jar hub
java -jar selenium-server-4.x.x.jar node --detect-drivers true
Step 5: Leverage New Features in Test Cases
Incorporate new features like relative locators, multi-tab support, and element-level screenshots into your test cases to optimize coverage and reliability.
Example:
from selenium.webdriver.support.relative_locator import locate_with
element_below = driver.find_element(locate_with(By.TAG_NAME, "button").below(input_field))
element_below.click()
Also read: Step-By-Step Guide To Selenium Automation Testing 2024
Why Selenium 4 Matters for Test Automation Companies
For Selenium Automation Testing Companies, Selenium 4 offers numerous advantages:
1. Enhanced Stability: With the adoption of the W3C WebDriver protocol, Selenium 4 ensures uniform browser behavior, eliminating compatibility issues across different browser versions and platforms. This enhanced stability allows companies to focus on building robust automation suites without worrying about inconsistencies caused by browser updates.
2. Reduced Maintenance Efforts: The simplified APIs in Selenium 4 streamline script maintenance. Features like relative locators and improved element interactions reduce the complexity of identifying dynamic elements, making test scripts easier to update and manage as application UIs evolve.
3. Advanced Debugging Tools: DevTools integration provides unparalleled access to browser-level information, enabling engineers to:
a. Monitor and intercept network requests.
b. Capture detailed logs for better diagnostics.
c. Profile application performance during test execution. These tools empower QA teams to debug issues faster, ensuring high-quality test outcomes.
4. Scalable Testing: Selenium Grid’s enhanced architecture supports massive parallel test execution, leveraging Docker containers and Kubernetes for scalability. By enabling seamless integration with cloud services like AWS and Azure, Selenium 4 allows test automation companies to scale their testing infrastructure efficiently and cost-effectively.
5. Improved Test Coverage: The addition of relative locators, multi-window support, and element-level screenshots expands the testing capabilities. Testers can now cover complex workflows, such as verifying dynamic layouts, testing multi-tab interactions, and capturing granular UI-level details for validation and reporting.
6. Future-Ready Framework: By embracing modern standards like W3C WebDriver and deprecating outdated methods, Selenium 4 positions itself as a future-ready automation framework. This forward-thinking strategy provides compatibility with future browser capabilities and integrations, thereby protecting enterprises’ test automation expenditures.
Conclusion
Selenium 4 is a major step forward in web automation, providing features that cater to the modern demands of Test Automation Companies and QA teams. By adopting Selenium 4, companies offering Selenium Testing Services can enhance their workflows, improve test stability, and scale their automation efforts efficiently.
Upgrading to Selenium 4 is not just about staying up to date – it’s about unlocking a new level of test automation capabilities for improved reliability and faster results. For organizations striving to optimize testing processes, Selenium 4 is an essential tool in their automation arsenal.
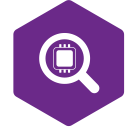
AutomationQA
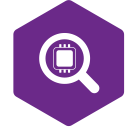
Latest posts by AutomationQA (see all)
- How AI is Enhancing Selenium, Cypress, and Playwright in Test Automation - March 11, 2025
- Playwright’s API Testing Capabilities: The New Frontier in Automation - March 4, 2025
- Cross-Browser Testing Made Easy: Exploring Playwright’s Unique Features - February 24, 2025