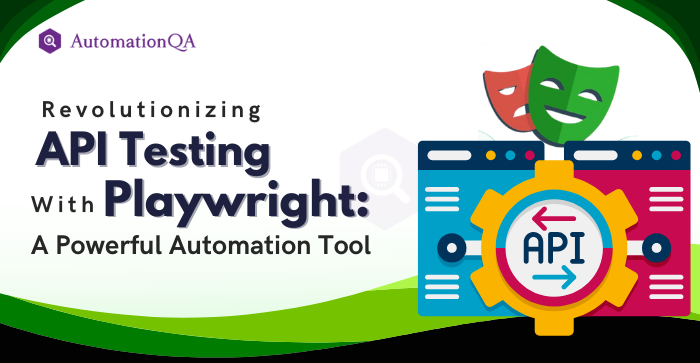
Automation testing has undergone a massive transformation in recent years. While Selenium has long been the industry standard for UI testing, new frameworks like Playwright are changing how developers approach end-to-end test automation.
Developed by Microsoft, Playwright is well-known for its strong browser automation features. However, its built-in API testing features are gaining traction as a powerful alternative to Postman, RestAssured, and Cypress API testing.
This article explores Playwright’s API testing capabilities, real-world use cases, best practices, and advanced features to help you integrate API testing seamlessly into your automation workflow.
Why API Testing Matters in Modern Development?
Before getting into Playwright’s API capabilities, it’s important to know why API testing is required.
- Speed and Efficiency: APIs act as the backbone of modern applications. Testing them ensures faster and more reliable development cycles.
- Decoupling UI from Testing: Unlike UI testing, API tests don’t depend on front-end elements, making them less prone to breakage.
- Early Bug Detection: API tests help catch integration problems before they affect end users.
- Scalability: With API tests, you can validate multiple endpoints simultaneously, making large-scale testing more manageable.
Many teams rely on tools like Postman, RestAssured, or Cypress for API testing. However, Playwright now provides a compelling alternative with its built-in API testing capabilities.
Let’s also discuss about the top Playwright automation testing features QA teams must use in 2025.
How Playwright Handles API Testing
Playwright’s API testing is designed to be simple yet powerful. It allows you to send HTTP requests, assert responses, and integrate API tests within a broader end-to-end testing framework.
1. Sending API Requests
Playwright includes an APIRequestContext, which enables developers to send HTTP requests directly from test scripts. You can send GET, POST, PUT, DELETE, and other HTTP methods seamlessly.
Example: Making a GET request:
const { request } = require('@playwright/test');
(async () => {
const apiContext = await request.newContext();
const response = await apiContext.get('https://jsonplaceholder.typicode.com/posts/1');
console.log(await response.json()); // Logs the response body
})();
This simple script fetches data from an API endpoint and logs the response.
2. Validating API Responses
Verifying responses is a core part of API testing. Playwright allows you to assert status codes, response bodies, headers, and timing.
Example: Validating status codes and response body:
const { test, expect, request } = require('@playwright/test');
test('Validate API response', async ({ request }) => {
const response = await request.get('https://jsonplaceholder.typicode.com/posts/1');
expect(response.status()).toBe(200); // Status code assertion
const data = await response.json();
expect(data.id).toBe(1); // Checking response body content
});
This test ensures that the response status is 200 and the returned data contains the expected values.
3. Handling Authentication
Many APIs require authentication via tokens, API keys, or OAuth. Playwright supports these mechanisms.
Example: API testing with Bearer Token Authentication:
test('Authenticated API request', async ({ request }) => {
const apiContext = await request.newContext({
extraHTTPHeaders: {
'Authorization': Bearer YOUR_ACCESS_TOKEN
}
});
const response = await apiContext.get('https://api.example.com/protected-resource'); expect(response.status()).toBe(200);
});
This approach ensures that authenticated endpoints can be tested easily.
4. Sending POST Requests with JSON Payloads
Playwright allows you to send requests with body data for POST, PUT, or PATCH operations.
Example: Making a POST request:
test('Create new resource via API', async ({ request }) => {
const response = await request.post('https://jsonplaceholder.typicode.com/posts', {
data: {
title: 'New Post',
body: 'This is a test post',
userId: 1
}
});
expect(response.status()).toBe(201);
const responseBody = await response.json();
expect(responseBody.title).toBe('New Post');
});
This test verifies that a new resource is created successfully.
5. API Mocking and Intercepting Requests
Playwright provides API request interception, allowing you to mock responses. This is useful for testing edge cases or simulating backend failures.
Example: Mocking API responses:
test('Mock API response', async ({ page }) => {
await page.route('https://api.example.com/data', route => {
route.fulfill({
status: 200,
contentType: 'application/json',
body: JSON.stringify({ message: 'Mocked response' })
});
});
await page.goto('https://example.com');
// Intercepted request will return the mocked response
});
This method is particularly useful for testing APIs in isolated environments.
Playwright vs. Other API Testing Tools
Playwright competes with established API testing tools like Postman, RestAssured, and Cypress. Here’s how it compares:
Feature | Playwright API Testing | Postman | RestAssured | Cypress API Testing |
---|---|---|---|---|
Built-in Automation | ✅ Yes (Integrated with UI testing) | ❌ No | ❌ No | ✅ Yes |
Code-Based Testing | ✅ JavaScript/TypeScript | ❌ GUI-Based | ✅ Java | ✅ JavaScript |
Mocking & Interception | ✅ Yes | ❌ No | ❌ No | ✅ Yes |
CI/CD Integration | ✅ Seamless | ✅ Yes | ✅ Yes | ✅ Yes |
Multi-Browser Support | ✅ Yes | ❌ No | ❌ No | ✅ Yes |
Playwright stands out for its ability to test APIs within a broader automation suite. If you’re already using Playwright for UI tests, leveraging it for API testing makes sense.
When to Use Playwright for API Testing
Playwright is a great choice for API testing in the following scenarios:
- End-to-End Testing – If you want to test UI interactions along with API validations.
- Mocking Backend APIs – When you need to simulate API behavior for frontend testing.
- CI/CD Pipelines – If you want to run API tests within a continuous integration workflow.
- Cross-Browser API Testing – If you need to verify API behavior across multiple environments.
However, if you’re working on extensive API-only test suites with heavy data-driven tests, traditional tools like Postman or RestAssured might be a better fit.
Final Thoughts
Playwright’s API testing capabilities add another dimension to its automation toolkit. It enables developers to write API tests with the same framework used for UI automation, reducing dependency on multiple tools.
With features like authentication handling, response validation, and request interception, Playwright is proving to be a powerful alternative to traditional API testing tools. Whether you’re testing web applications, APIs, or both, integrating Playwright can improve test efficiency and reliability.
If you haven’t explored Playwright’s API testing yet, now is the time. It might just be the automation solution your team needs.
Would you like a hands-on guide on setting up Playwright for API testing? Contact us now!
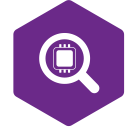
AutomationQA
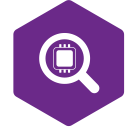
Latest posts by AutomationQA (see all)
- How to Use Cypress to Test Web Applications for Accessibility Compliance - April 9, 2025
- The Revolutionary Effect of Automation Driven by AI on QA Software Testing - April 3, 2025
- AI-Powered Self-Healing Test Automation for Mobile Apps - March 27, 2025