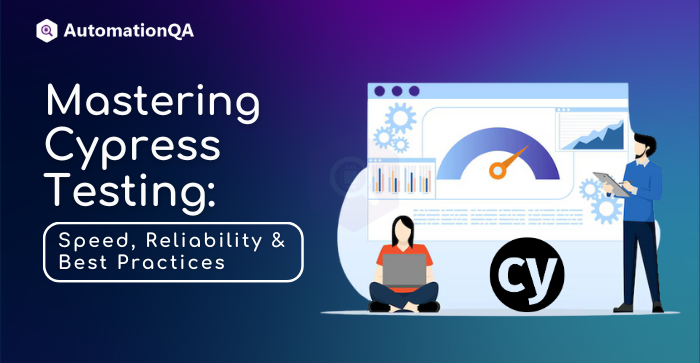
What if a critical feature on your website breaks after a new update; without you realizing it until users start complaining?
E2E testing ensures seamless application workflows, preventing hidden issues in performance, security, or usability. Cypress for UI testing streamlines automation with fast execution, real-time debugging, and automatic waiting, overcoming challenges seen in Cypress vs Selenium for testing.
However, slow and flaky tests can delay deployments and reduce developer confidence. Optimizing Cypress testing tips enhances speed, reliability, and CI/CD efficiency, enabling faster Cypress tests, high-quality releases.
The following sections will cover Cypress automation best practices, optimization techniques, and advanced strategies to make Cypress automation services more reliable and easier to maintain.
You might also want to read about why Cypress is the preferred automation tool.
Setting Up Cypress for E2E Testing
Before diving into writing test cases, it is essential to set up Cypress for UI testing correctly to ensure a smooth development and testing experience. A well-configured Cypress environment enhances test stability, execution speed, and maintainability. This section covers installation, folder structure, and CI/CD integration, providing a solid foundation for Cypress automation services.
Installation and Configuration
Setting up Cypress is straightforward, thanks to its developer-friendly installation process. It requires minimal configuration, making it an excellent choice for teams looking to automate testing without a steep learning curve.
Installing Cypress
Cypress can be installed using either npm or yarn. Open a terminal and run:
npm install cypress --save-dev
Or
yarn add cypress –dev
After installation, open Cypress using:
npx cypress open
This launches the Cypress Test Runner, where you can create and run test cases.
Basic Configuration
Cypress stores its configuration in either cypress.json (for older versions) or cypress.config.js (for Cypress 10+). Common configurations include:
- Setting base URLs:
{
"baseUrl": "http://localhost:3000",
"viewportWidth": 1280,
"viewportHeight": 720
}
- Enabling retries to handle flaky tests:
{
"retries": {
"runMode": 2,
"openMode": 1
}
}
- Adjusting these settings ensures faster test execution and reduces flakiness.
Folder Structure and Best Practices
A well-organized test directory helps maintain clarity and ensures tests are easy to read and scale. Cypress follows a default folder structure:
- cypress/integration/ → Stores actual test cases (.spec.js or .cy.js files).
- cypress/fixtures/ → Contains test data, mock responses, and JSON files.
- cypress/support/ → Holds reusable custom commands and configurations.
- cypress/plugins/ → Used for extending Cypress functionality.
Best Practices for Folder Organization:
- Keep tests modular: Separate test cases based on features (e.g., login.spec.js, checkout.spec.js).
- Use meaningful test names for clarity.
- Store reusable functions in cypress/support/commands.js for easy test maintenance.
Integrating Cypress with CI/CD Pipelines
Automating Cypress tests within CI/CD pipelines ensures faster feedback and maintains code quality. Popular CI/CD tools like GitHub Actions, Jenkins, and CircleCI can seamlessly integrate Cypress.
- Running Cypress tests in CI:
npx cypress run
- Setting up Cypress in GitHub Actions:
jobs:
cypress-test:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Install dependencies
run: npm install
- name: Run Cypress tests
run: npx cypress run
For consistent testing environments, running Cypress in Docker containers prevents dependency-related issues:
docker run -it -v $PWD:/e2e -w /e2e cypress/included:latest
Integrating Cypress within CI/CD ensures reliability, automating the execution of tests every time new code is pushed.
Writing Effective Cypress Tests
Once Cypress is set up, the next step is to write clean, structured, and maintainable tests. Poorly written tests lead to flakiness and difficulty in debugging, which slows down development.
Writing Testable Code
Well-structured applications are easier to test. A key principle of testable UI code is using stable selectors:
- Avoid: Targeting elements by CSS class names (which may change).
- Use: data-testid or data-cy attributes for more resilient selectors
cy.get('[data-cy="submit-button"]').click();
Structuring Tests
Cypress follows the Mocha test framework, allowing tests to be structured with:
- describe → Groups related tests.
- it → Defines an individual test case.
- beforeEach → Runs setup code before each test.
Example:
describe('Login Page Tests', () => {
beforeEach(() => {
cy.visit('/login');
});
it('Should log in with valid credentials', () => {
cy.get('[data-cy="username"]').type('user123');
cy.get('[data-cy="password"]').type('password');
cy.get('[data-cy="login-button"]').click();
cy.url().should('include', '/dashboard');
});
});
Writing Assertions
Assertions validate that expected behavior occurs. Cypress provides:
- Implicit assertions:
cy.get('h1').should('contain', 'Welcome');
- Explicit assertions:
cy.get('h1').then(($el) => {
expect($el.text()).to.include('Welcome');
});
Handling Asynchronous Code
Cypress automatically waits for elements, removing the need for manual delays. However, network requests sometimes require additional handling:
- Bad practice (hardcoded waits)
cy.wait(5000); // Avoid this!
- Best practice (intercept API responses):
cy.intercept('POST', '/api/login').as('loginRequest');
cy.wait('@loginRequest').its('response.statusCode').should('eq', 200);
Best Practices for Cypress Testing
A well-structured and optimized Cypress test suite improves automation reliability, speeds up execution, and reduces maintenance overhead. Follow these best practices to ensure efficient Cypress testing.
Folder Organization & Test Modularity
Organizing test files correctly keeps them maintainable and scalable. Use a structured hierarchy:
- cypress/integration/ → Stores feature-specific test cases (login.spec.js, checkout.spec.js).
- cypress/fixtures/ → Contains reusable test data in JSON format.
- cypress/support/ → Holds custom commands and reusable functions.
- cypress/plugins/ → Extends Cypress functionality (e.g., database handling).
- Keep test files modular. Each test case should focus on a single feature to simplify debugging and prevent dependencies.
Using Cypress Fixtures for Test Data
Avoid hardcoded test data by using Cypress fixtures. Store data in JSON files and reference them dynamically in tests:
cy.fixture('user.json').then((user) => {
cy.get('[data-cy="username"]').type(user.username);
cy.get('[data-cy="password"]').type(user.password);
});
Fixtures improve reusability and make tests independent of static inputs.
Creating Custom Cypress Commands
Reduce repetitive steps by defining reusable commands in cypress/support/commands.js:
Cypress.Commands.add('login', (username, password) => {
cy.get('[data-cy="username"]').type(username);
cy.get('[data-cy="password"]').type(password);
cy.get('[data-cy="login-button"]').click();
});
Now, login can be called in tests with:
cy.login('user123', 'password');
This keeps tests clean and improves maintainability.
Running Tests in Parallel for Faster Execution
Parallel execution speeds up test runs by distributing them across multiple machines or CI workers. Enable parallelization in CI/CD:
npx cypress run --parallel
Also, split large test files into smaller independent suites to improve execution time.
Avoiding Flaky Cypress Tests: Best Practices & Common Pitfalls
Flaky tests fail inconsistently due to poor waiting strategies, unstable selectors, or dynamic content.
Understanding Flaky Tests & Their Causes
Common causes include:
- Poorly chosen selectors (e.g., using CSS class names that change frequently).
- Tests depending on unpredictable network responses.
- Hardcoded wait times (cy.wait(5000)) instead of dynamic waiting.
Using Cypress’s Built-in Waiting & Debugging Features
Cypress automatically waits for elements but adding explicit waits improves test reliability:
Correct approach:
cy.get('[data-cy="submit-button"]').should('be.visible').click();
Incorrect approach:
cy.wait(5000);
cy.get('[data-cy="submit-button"]').click();
Use Cypress’s built-in debugging tools (.debug(), .pause()) to inspect test failures.
Avoiding Over-Complex Test Cases
Each test should validate a single feature rather than covering multiple flows.
- Break down large tests: Instead of testing login + checkout in one test, separate them.
- Use describe blocks to logically group tests for better readability.
Ensuring Proper Test Isolation
Tests should not depend on previous tests.
- Use beforeEach() to reset application state before every test.
- Clear cookies and local storage to prevent data leakage:
beforeEach(() => {
cy.clearCookies();
cy.clearLocalStorage();
});
This ensures each test starts fresh.
Managing Test Stability & Dependencies in Cypress
Many applications dynamically update elements after API calls. Instead of waiting for a fixed time, intercept API responses:
cy.intercept('POST', '/api/login').as('loginRequest');
cy.wait('@loginRequest').its('response.statusCode').should('eq', 200);
This ensures Cypress interacts only when the request is complete.
Use before(), beforeEach(), and afterEach() hooks to manage setup and cleanup:
beforeEach(() => {
cy.visit('/login');
});
This avoids duplication and keeps tests organized.
Instead of hardcoding credentials, use environment variables in cypress.env.json:
{
"username": "admin",
"password": "securepassword"
}
Retrieve them in tests:
cy.get('[data-cy="username"]').type(Cypress.env('username'));
This keeps tests adaptable across environments (dev, staging, production).
Cypress vs. Selenium: Key Differences
Feature | Cypress | Selenium |
---|---|---|
Test Execution | Faster (runs inside browser) | Slower (uses WebDriver) |
Setup | Simple (npm install cypress) | Requires drivers, configurations |
Debugging | Built-in time travel & snapshots | Requires manual debugging |
Automatic Waiting | Yes | No (needs explicit waits) |
Cross-Browser Support | Chrome, Edge, Firefox | All major browsers, including Safari |
Mobile Testing | No | Yes |
Choose Cypress for fast UI testing and developer-friendly debugging. Use Selenium when extensive cross-browser or mobile testing is required.
Advanced Cypress Techniques
For enterprise projects:
- Organize tests using a domain-driven structure.
- Implement API mocking to avoid reliance on unstable backend responses.
- Use test tagging to categorize critical vs. non-critical tests.
Optimizing CI/CD Execution
To enhance CI/CD performance:
- Run Cypress tests headless in CI
- npx cypress run –headless
- Enable test artifacts (screenshots/videos) for debugging failures.
- Cache dependencies (node_modules, Cypress binaries) to speed up test runs.
Avoiding Common Test Automation Mistakes
- Not using unique selectors → Use data-cy attributes instead of generic classes.
- Testing too much in one case → Keep tests atomic and focused.
- Ignoring API response validation → Always validate API calls before asserting UI changes.
- Not cleaning test state → Reset storage and cookies between tests to prevent flaky behavior.
Final Thoughts
Optimizing Cypress automation services requires structured organization, reusable patterns, and reliable waiting strategies. Following these Cypress automation best practices will improve test execution speed, reduce flakiness, and ensure high-quality test automation.
By integrating Cypress for UI testing effectively in CI/CD, adopting modular test structures, and leveraging Cypress testing tips, teams can maintain faster Cypress tests and ensure scalable Cypress automation services.
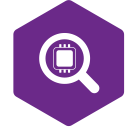
AutomationQA
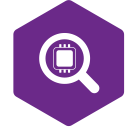