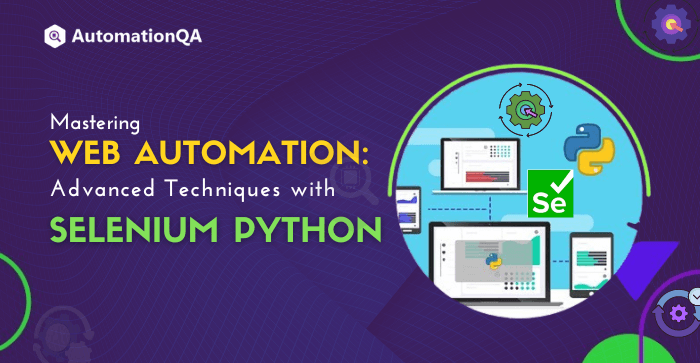
Are you struggling to write and maintain your complex application’s web automation test script? If yes, then you are not alone; many businesses face these difficulties due to the dynamic nature of their web applications. Further, frequent updates and cross-platform compatibility worsen the situation. This is why testing companies prefer the powerful combination of Python and Selenium to overcome challenges. This combo enables testers and developers to connect with web applications more efficiently.
Selenium and Python act like secret tools, enabling smooth and sophisticated interactions. While basic Selenium commands serve as the entry point, the advanced features unlock hidden capabilities within web applications. They transform your web automation testing into remarkable feats. Let’s further explore this powerful technique and discuss leveraging it for your benefit. Everything you need to know will be covered in this blog, from configuring the testing environment to writing your first line of code. It will help you elevate your app testing to new heights.
Setting Up Selenium with Python for Automation Testing
Setting Up The Environment
Establishing a Selenium environment with Python involves installing Python, Selenium, and WebDrivers to ensure seamless integration and execution. Follow these steps to guarantee that everything is correctly configured for seamless development and testing with Selenium Python.
Installing Python
1. Visit the [official Python website]
(https://www.python.org) to obtain the most recent Python version for your operating system.
2. Launch the downloaded installer and follow the instructions.
3. check the "Add Python to PATH"
box during installation.
4. To check the installation, open a command prompt or terminal and enter ‘python -version.’ The installed Python version should be displayed.
Installing Selenium
1. Open a command prompt or terminal.
2. Run the following command to install Selenium using pip:
"'sh pip install Selenium ''
Pip downloads and installs the Selenium library and its dependencies.
Installing WebDrivers
Automation testing companies understand that Selenium requires distinct WebDrivers to connect with various browsers. As a result, they download and install the proper WebDriver for the browser they want to automate. The most common browsers companies use with Selenium are Firefox, Chrome, and Safari. Below, we have demonstrated how to install ChromeDriver for Selenium.
Installing ChromeDriver For Selenium
1. Visit the [ChromeDriver download page] (https://sites.google.com/a/chromium.org/chromedriver/downloads)
2. Download the ChromeDriver version that is compatible with the version of Google Chrome you have installed.
3. Extract the downloaded file.
4. Place the extracted chromedriver executable in a directory in your system’s PATH variable. It helps testing automation companies avoid script failure as inconsistent behavior in the case of multiple versions.
Writing Your First Selenium Script
In this part, we’ll show you how to construct your first Selenium testing script with Python.
Run the script on the local setup before moving on to the following parts. It will ensure your environment is correctly configured, and you can execute Selenium scripts using Python.
Here’s a simple example script:
"`Python
from selenium import web driver
Create a new instance of the Chrome driver.
driver = webdriver.Chrome()
Navigate to a website.
driver.get("https://www.example.com")
Find an element in Selenium testing services by its name attribute
element = driver.find_element_by_name("example_name")
print(element.text)
Close the browser
driver.quit()
```
Save this script as `first_test.py` and run it using the command:
"`sh
python first_test.py
```
If everything is set up successfully, the script will launch Chrome, go to the supplied URL, locate an element, print its text, and exit the browser.
You are now ready to start your Selenium automation journey using Python!
Must-Known Advanced Actions For Complex Applications
Basic Selenium commands are best used for simple interactions such as clicking buttons and entering text. However, real-world online applications frequently require more sophisticated automation. As a result, Selenium Testing Companies employ advanced actions when handling dynamic information, waiting for certain conditions, or dealing with complex user interactions.
Let’s look into seven advanced Selenium actions with practical examples.
Advanced Actions with Code Examples
Handling Dynamic Elements
Locating and interacting with dynamically appearing or disappearing objects on a web page.
Scenario
Consider a form that automatically adds new input fields based on user decisions. Using basic instructions may result in discovering non-existent items. Instead, use advanced actions to await the arrival of dynamic elements.
"`Python
from Selenium.webdriver.common.by import By
from Selenium.webdriver.support.ui import WebDriverWait
from Selenium.webdriver.support import expected_conditions as EC
element = WebDriverWait(driver, 10).until(
EC.presence_of_element_located((By.ID, "dynamicElementID"))
)
element.click()
```
Working with Frames
Selenium automation companies may switch between web page frames to perform actions in specific contexts.
Scenario
You discover a webpage with numerous frames; your script must interact with components within these frames. Basic Selenium commands will not work outside of the preset content. Advanced actions enable you to move contexts seamlessly.
"`python
driver.switch_to.frame("frameName")
# Perform actions inside the frame
driver.switch_to.default_content()
```
Mouse Actions – Hover
Simulating mouse hovering allows you to interact with only visible components when you hover.
Scenario
You have a navigation menu that only shows extra options when hovering over. Basic commands need to include these elements. Selenium automation testing company uses advanced actions, like mouse hovering, to ensure accurate interactions.
"`Python
from Selenium.webdriver.common.action_chains import ActionChains
element_to_hover_over = driver.find_element(By.ID, "hoverElementId")
hover = ActionChains(driver).move_to_element(element_to_hover_over)
hover.perform()
```
Handling Alerts
Manage pop-up alerts and confirm or dismiss them.
Scenario
Before submitting a form, the user receives an alert requesting confirmation. Basic Selenium commands will not handle alerts, and advanced actions are required to deal with pop-ups.
```python
alert = driver.switch_to.alert
Accept the alert
alert.accept()
Reject the alert
alert.dismiss()
Send text to the alert prompt
alert.send_keys("Your Text Here")
```
Keyboard Actions
Simulating keyboard actions to perform particular key-related tasks during web automation testing.
Scenario
When automating testing, you may need to imitate keyboard activities such as key presses or key combinations.
"`Python
from Selenium.webdriver.common.keys import Keys
# Sending keys to an input field
text_field = driver.find_element_by_id("text-field-id")
text_field.send_keys("Hello, World!")
# Combining keys (e.g., Ctrl+A to select all text)
text_field.send_keys(Keys.CONTROL + "a")
```
Executing JavaScript
Running custom JavaScript code to alter the DOM or carry out particular operations.
Scenario
You encounter a simple click that doesn’t trigger the expected behavior. Advanced actions, such as invoking JavaScript, provide a more versatile solution.
"`Python
r_text = driver.execute_script(“return arguments[0].innerText;”, element)
driver.execute_script("window.scrollTo(0, document.body.scrollHeight);")
element = driver.find_element_by_id("yourElementId")
inne
“`
Advanced Waits
Before interacting with web items in Selenium Python, add custom wait conditions to ensure they are clickable, visible, and so on.
Scenario
Your automation script should click a button that displays after a delay or when a specific condition is met. Basic commands may fail in this scenario—advanced waits to verify synchronization with the webpage.
"`Python
from Selenium.webdriver.common.by import By
from Selenium.webdriver.support.ui import WebDriverWait
from Selenium.webdriver.support import expected_conditions as EC
element = WebDriverWait(driver, 10).until(
EC.element_to_be_clickable((By.ID, "someElementID"))
)
element.click()
```
Browser Navigation
Navigate the browser history by going back, forward, or refreshing the page.
Scenario
After submitting a form, automation testing companies should return to the previous page to check for any changes the firm might have triggered. Basic commands can handle the navigation back to the last page. Still, advanced navigation actions are preferred for more precise control and reliability.
"`Python
driver.back()
driver.forward()
driver.refresh()
```
Advanced Techniques and Best Practices
These advanced strategies will help you improve your ability to use Selenium with Python. These advanced techniques aim to enhance your testing efficiency and effectiveness.
Page Object Model (POM)
Organize your Selenium scripts using the Page Object Model (POM) architectural pattern. Leading test automation companies use this method to increase code maintainability and readability.
Data-Driven Testing
Modify your script tests to work with a variety of input datasets. This practice enhances test coverage and efficiency, allowing you to validate functionality across different scenarios.
Parallel Testing
use Python’s multiprocessing or threading modules to perform tests across multiple browsers or environments. This technique reduces the time required to execute your tests, improving productivity.
Logging and Reporting
Integrate logging frameworks like Log4j or custom Python logging modules into your testing system. Detailed test execution logs help create thorough test reports, aiding in troubleshooting and analysis.
Conclusion
Embracing Selenium with Python frameworks is a game-changer for advanced web automation. These frameworks give the organization, scalability, and efficiency required to negotiate the intricacies of modern web applications. Whether you opt for Robot Framework’s keyword-driven simplicity or Behave’s BDD approach, integrating these frameworks into your Selenium workflow empowers you to build and maintain robust automation scripts.
Leveraging these advanced techniques can enhance your testing capabilities and ensure thorough and reliable automation. This approach simplifies your testing process and significantly reduces maintenance overhead. Elevate your web testing capabilities and journey towards more efficient and reliable automation with Selenium Python frameworks. Happy testing!
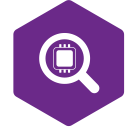
AutomationQA
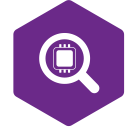
Latest posts by AutomationQA (see all)
- AI-Powered Self-Healing Test Automation for Mobile Apps - March 27, 2025
- How to Boost Your Cypress Testing with Component and Parallel Execution - March 21, 2025
- Migrating Test Suites to Modern Testing Frameworks: A Comprehensive Guide - March 18, 2025